Debugging is crucial in app development to catch issues and optimize performance. When developing in Android Studio, adding debug symbols allows developers to view more detailed stack traces, understand crash logs better, and gain valuable insights into the app’s behavior.
In this guide, we’ll cover everything from the basics to more advanced methods of adding debug symbols in Android Studio, all tailored to ensure any developer can follow along with ease.
Table of Contents
1. What Are Debug Symbols?
- Definition: Debug symbols are metadata that map the compiled code back to the original source code. This mapping allows tools to provide more human-readable information during debugging sessions.
- Importance: Without debug symbols, crash logs and stack traces might only show memory addresses instead of useful function names and line numbers. This makes tracking issues much more complex.
- Use Case Scenarios: Debug symbols are crucial for identifying issues, optimizing performance, and improving code readability in testing environments.
2. Overview of Debugging in Android Studio
- Purpose of Android Studio’s Debugging Features: Android Studio provides built-in tools to add and utilize debug symbols, which improve crash analysis and troubleshooting capabilities.
- Supported Symbol Types:
- Java and Kotlin: Android Studio natively supports symbols for Java and Kotlin, making debugging straightforward.
- Native Code (C/C++): For native modules using C++ or other native libraries, Android Studio allows the addition of debug symbols to further improve stack trace accuracy.
- Challenges Without Debug Symbols: With no debug symbols, crash reports show vague, low-level memory addresses, which offer little guidance on root issues. Adding debug symbols overcomes these challenges by providing detailed information.
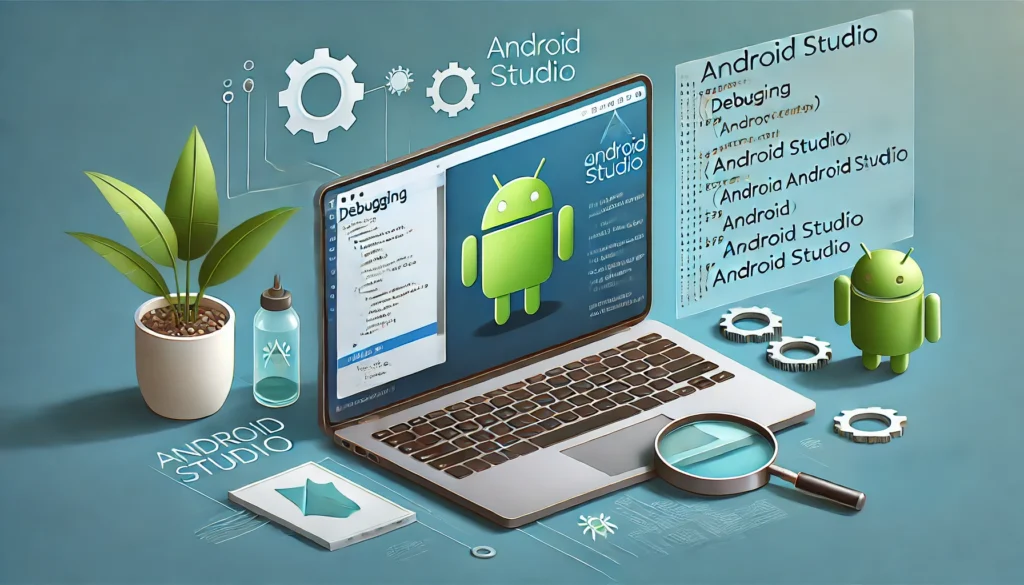
3. Adding Debug Symbols in Android Studio
This section provides a detailed step-by-step guide to adding debug symbols for both Java/Kotlin and Native (C++) code in Android Studio.
Step 1: Setting Up a Debuggable Build
- Debuggable Flag in
build.gradle
: To add debug symbols, the app must be in debug mode. Inbuild.gradle
:
android {
buildTypes {
debug {
debuggable true
}
}
}
- Explanation of Build Types: Distinguish between
debug
andrelease
builds.debug
builds are for internal testing and include symbols, whilerelease
builds prioritize optimization and often strip out debug information.
Step 2: Enabling ProGuard (Optional for Debug Builds)
- Why ProGuard Matters: ProGuard is a code obfuscation tool for Android that removes unused code, renames classes and variables, and optimizes bytecode. It’s typically used in release builds but can also be helpful in debugging by minimizing code complexity.
- Keeping Debug Symbols with ProGuard:
- Enable ProGuard by adding this to
build.gradle
:
- Enable ProGuard by adding this to
buildTypes {
release {
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
Retaining Symbols: In proguard-rules.pro
, add:
-keep class com.example.yourapp.** { *; }
-keepattributes *Annotation*
- This ensures ProGuard won’t strip away crucial debugging information.
Step 3: Adding Native Debug Symbols (For C++/NDK Code)
- NDK Integration: If your app uses the Native Development Kit (NDK) for C++ or other native languages, Android Studio can add symbols for these libraries.
- Enabling Debug Symbols:
- In your
CMakeLists.txt
orndk-build
settings, ensure debug symbols are generated by setting:
- In your
set(CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -g")
abiFilters
for Target Architectures: Specify the CPU architectures for which you’re generating symbols, such as armeabi-v7a
and x86_64
. This can be set in build.gradle
:
android {
defaultConfig {
ndk {
abiFilters "armeabi-v7a", "x86_64"
}
}
}
4. Generating and Accessing Symbol Files
- Symbol Files Location:
- When you compile your app, symbol files are typically generated in
app/build/intermediates/symbols/<buildVariant>
.
- When you compile your app, symbol files are typically generated in
- Understanding
.so
Files: For native libraries,.so
(shared object) files are where debug symbols reside. These files should be included in debugging builds but omitted from release builds if optimizing for performance.
5. Uploading Symbols to Google Play Console for Crash Reports
- Why Upload Symbols?: Uploading debug symbols to the Google Play Console enhances stack traces in crash reports, allowing you to view human-readable logs.
- Uploading ProGuard Mappings:
- After enabling ProGuard, you’ll have a
mapping.txt
file inapp/build/outputs/mapping/release
. - Google Play Console Integration:
- In the Google Play Console, navigate to Release Management > App Bundle Explorer.
- Upload
mapping.txt
for each release.
- After enabling ProGuard, you’ll have a
- Uploading Native Symbols (Symbols.zip): Generate a
symbols.zip
file with all.so
files for native code debugging, then upload this file in the App Bundle Explorer for better native crash logs.
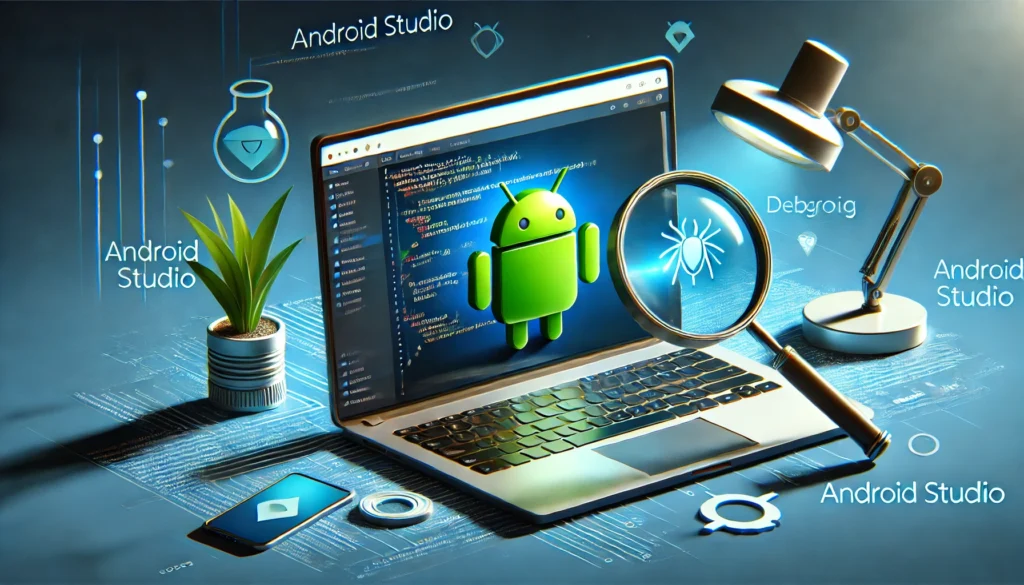
6. Debugging with Added Symbols in Android Studio
- Using Logcat: In Android Studio, the Logcat tab displays runtime logs. With debug symbols, stack traces will map to specific methods and line numbers.
- Breakpoints: Set breakpoints within methods. With debug symbols, you’ll see precise variable values and flow control.
- Debugger Window: Android Studio’s Debugger window gives a live view of variable values and control flow, enhanced by debug symbols.
7. Troubleshooting Common Issues
- Missing Debug Symbols: Sometimes debug symbols are missing due to build configuration issues. Ensure your
build.gradle
is correctly set up withdebuggable true
. - ProGuard Interference: ProGuard may strip symbols unintentionally if not configured correctly. Revisit ProGuard rules to ensure critical symbols are retained.
- Symbol File Not Recognized: Ensure you’re using the correct symbol files (mapping.txt for Java/Kotlin and symbols.zip for native libraries) and that they match the correct app version.
8. Advanced Tips for Efficient Debugging
- Using Filters in Logcat: Filter logs by tags or severity to focus on key issues.
- Network and Memory Debugging: Utilize tools like Network Profiler and Memory Profiler in Android Studio to complement debugging symbols and gain more insights.
- Real-Device Testing: Emulators are useful, but testing on real devices can reveal additional issues related to hardware, performance, and configuration.
9. FAQs on Debug Symbols in Android Studio
- Why Do My Stack Traces Still Look Obscure? Common reasons include incomplete symbol files or ProGuard stripping too many symbols. Ensure mappings and symbol files are correctly configured.
- How Can I Verify if Debug Symbols Are Working? In Logcat, if method names and line numbers are visible, the symbols are correctly integrated.
- Do I Need to Add Symbols for Each Build Variant? Yes, especially for variants that differ significantly, as they may have unique crash data.
10. Optimizing Debugging Workflow with Debug Symbols
Adding debug symbols in Android Studio is essential for understanding and troubleshooting app crashes efficiently. By following these steps and best practices, you can gain deeper insights into your app’s behavior and fix issues faster. With both Java/Kotlin and native debugging covered, Android Studio’s powerful tools combined with debug symbols can empower your development process for a more stable and optimized application.
frequently asked questions (FAQs) related to “Adding debug symbols in Android Studio“:
1. Why do my stack traces still look obscure even after adding debug symbols?
This issue can occur if the debug symbols are incomplete or missing for certain parts of the code. It may also happen if ProGuard or another obfuscation tool is stripping away essential information. Make sure that your symbol files (such as mapping.txt
for Java/Kotlin or symbols.zip
for native libraries) are generated correctly and that you’re uploading the right files.
2. How can I verify if debug symbols are working correctly?
In Android Studio, open Logcat after running your app in debug mode. If the symbols are correctly integrated, stack traces should display specific method names, class names, and line numbers instead of memory addresses. Setting breakpoints in the code and examining variable states can also confirm that symbols are working as expected.
3. Do I need to add debug symbols for each build variant?
Yes, each build variant may have unique crash data and requirements. Ensure that debug symbols are generated and uploaded for each relevant variant, especially if they differ significantly (e.g., debug
vs. release
builds or different API targets).
4. Can I add debug symbols for native code (C++) in Android Studio?
Yes, debug symbols can be added for native libraries built with C++ using the NDK. Enable symbols by adding -g
to the CMAKE_CXX_FLAGS
in your CMakeLists.txt
file or adjusting ndk-build
settings. This allows for clearer stack traces in crash logs for native code, especially when analyzing them on platforms like Google Play Console.
5. What should I do if the Google Play Console doesn’t recognize my symbol files?
First, double-check that the symbol files you are uploading correspond to the correct app version and architecture. If you’re uploading mapping.txt
for Java/Kotlin code or symbols.zip
for native libraries, ensure these files are placed correctly in your project structure and compiled accurately.