Understanding the classpath is essential for developing Android applications. In Android Studio, the classpath specifies where to find libraries and other resources necessary for compiling and running your application.
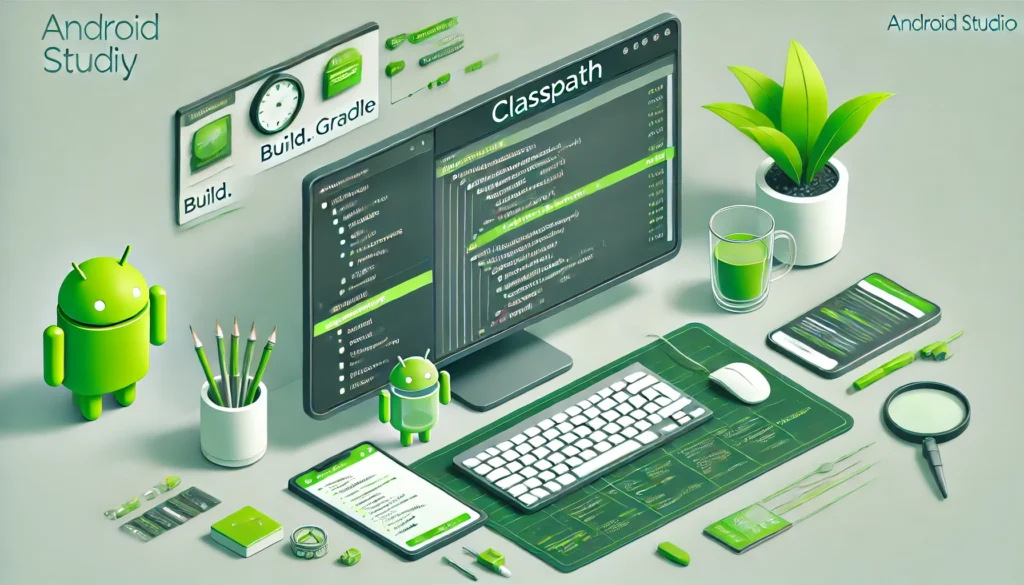
When configuring a new project, setting up dependencies, or troubleshooting, knowing where to add and manage the classpath is crucial. In this guide, we’ll walk through each step involved in configuring the classpath in the latest version of Android Studio, making it accessible even for beginners.
Table of Contents
Where to Add Classpath in New Android Studio
1. What is the Classpath in Android Studio?
- Overview of Classpath in Java and Android Development: The classpath is a parameter that tells the Java compiler and runtime where to find the required libraries and packages.
- Classpath in Build Systems: In Android development, we often use Gradle as our build system, which relies on the classpath to locate dependencies and plugins. This is generally managed in the
build.gradle
files in Android Studio.
2. Why Setting the Classpath Correctly is Important
- Incorrect configuration can lead to errors such as missing classes, compilation issues, or runtime errors.
- Proper classpath setup allows Android Studio to access external libraries and plugins, making it easier to develop robust applications.
Step 1: Understanding Gradle in Android Studio
Android Studio uses Gradle as its build automation tool, which is crucial for managing dependencies, organizing builds, and setting the classpath. When you open a project in Android Studio, you’ll notice two build.gradle
files:
- Project-level
build.gradle
: This file configures the global build settings for your entire project. - App-level
build.gradle
: This file defines settings specific to your app module, such as the dependencies and versions used in your app.
The classpath entry, especially for plugins, is usually defined in the Project-level build.gradle
file. The App-level file handles dependencies within the application itself.
Step 2: Adding the Classpath in Android Studio
Step-by-Step Guide for Beginners:
- Open Android Studio: Make sure your project is loaded and visible in the Project Explorer on the left-hand side.
- Locate the Project-Level
build.gradle
File:- In the Project Explorer, expand the folder with your project’s name.
- Look for the file labeled
build.gradle (Project: <Your Project Name>)
. This is the project-level file.
- Add the Classpath Entry:
- Open the
build.gradle
file for editing by double-clicking on it. - Locate the
dependencies
block within this file. - Add the classpath entry here. For example, if you’re adding the classpath for the Google services plugin, your code would look like this:
- Open the
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:7.0.0'
classpath 'com.google.gms:google-services:4.3.8' // Add classpath here
}
}
- Sync the Project:
After adding your classpath, click “Sync Now” in the notification bar at the top of the editor. This step is essential because it applies your changes and updates the build configuration.
Additional Example:
Suppose you need to add a classpath for the AndroidX Navigation library; you would add it similarly in the dependencies block.
Step 3: Understanding App-Level Dependencies
Once you’ve added classpaths at the project level, you might also need to add specific libraries or plugins at the app level.
Navigate to the App-Levelbuild.gradle
File:
In the Project Explorer, look forbuild.gradle (Module: app)
.
Adding Dependencies:
Open this file and locate thedependencies
block.
Here, you can add libraries needed for your app, such as:
dependencies {
implementation 'androidx.navigation:navigation-fragment:2.3.5'
implementation 'androidx.navigation:navigation-ui:2.3.5'
}
- Sync and Verify:
- After adding dependencies, sync the project to ensure everything is configured correctly.
Step 4: Common Issues and Troubleshooting Classpath Errors
1. Common Classpath Errors:
- “Failed to resolve” error: This can happen if the classpath dependency is incorrect. Double-check the dependency version and the repository.
- “Plugin not found” error: Make sure you’ve specified the correct plugin in the classpath.
- Sync Issues: If Android Studio fails to sync, try cleaning and rebuilding the project via Build > Clean Project and Build > Rebuild Project.
2. Clearing the Gradle Cache:
- If you encounter persistent errors, clearing the Gradle cache can help. Go to File > Invalidate Caches / Restart and select “Invalidate and Restart.”
Step 5: Additional Tips for Classpath Management
- Keep Dependencies Updated: Gradle dependencies are frequently updated, and using the latest versions can avoid compatibility issues.
- Use Gradle Plugin Version Compatibility Guide: Refer to the Android Gradle Plugin Version Compatibility Guide to ensure compatibility between your Gradle version and Android Studio.
Properly managing the classpath in Android Studio is essential for maintaining a stable development environment. By following these steps, you’ll be able to add and manage classpaths effectively in the latest Android Studio version, enabling you to build your applications without unexpected build issues.
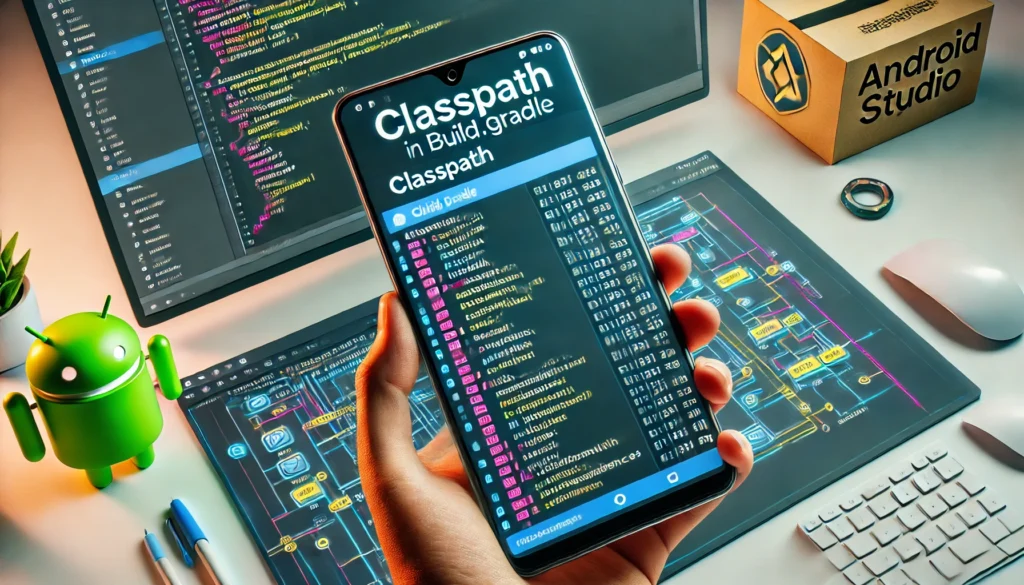
frequently asked questions (FAQs) for a guide on adding classpath in Android Studio, especially helpful for beginners.
1. What is the classpath in Android Studio, and why is it important?
The classpath in Android Studio is a setting that defines where the system should look for compiled code, libraries, and plugins necessary to build and run an Android application. Setting the correct classpath ensures that Android Studio can access these resources, preventing build errors and enabling smooth project execution.
2. Where exactly do I add the classpath in Android Studio?
In Android Studio, the classpath for plugins is usually added to the Project-level build.gradle
file. You can find this file by expanding your project in the Project Explorer and locating build.gradle
(Project). Inside this file, add the classpath entry in the dependencies
block under buildscript
.
3. What’s the difference between the Project-level and App-level build.gradle
files?
The Project-level build.gradle
file is used for project-wide configuration, including setting up plugins and classpaths needed across the entire project. The App-level build.gradle
file (found in the app
module) is specific to the app module and manages dependencies and configurations that apply directly to your app.
4. How do I fix the “Failed to resolve” error when adding a classpath?
The “Failed to resolve” error usually indicates an incorrect classpath dependency or missing repository. Double-check that you’ve typed the classpath correctly and that you’ve included the necessary repositories (like google()
and mavenCentral()
) in the build.gradle
file.
5. How can I update or remove a classpath in Android Studio?
To update a classpath, open the Project-level build.gradle
file and modify the classpath dependency to the new version. To remove a classpath, simply delete its line from the dependencies
block. After making changes, click “Sync Now” to apply the updates.